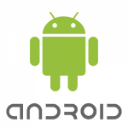
端末の起動完了を検知したいことがあります。
そのような場合には BroadcastReceiver で
OS の出しているイベントを受け取る必要があります。
結構基本中の基本ですが記事として書いたことがなかったので書いておきます。
public class BootCompletedReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent){ if(Intent.ACTION_BOOT_COMPLETED.equals(intent.getAction())){ // Boot completed! } } }
<receiver android:name=".BootCompletedReceiver" android:permission="android.permission.RECEIVE_BOOT_COMPLETED" > <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED" /> </intent-filter> </receiver>
毎回書くのも微妙な気がするので BootCompletedReceiver を作ってみたけどいらないかも?
/** * <h1>Usage</h1> * * <h2>Java</h2> * * <pre> * public class YourBootCompletedReceiver extends BootCompletedReceiver { * @Override * public void onBootCompleted(){ * // your code here * } * } * </pre> * * <h2>AndroidManifest.xml</h2> * * <pre> * <receiver * android:name=".YourBootCompletedReceiver" * android:permission="android.permission.RECEIVE_BOOT_COMPLETED" > * <intent-filter> * <action android:name="android.intent.action.BOOT_COMPLETED" /> * </intent-filter> * </receiver> * </pre> */ public abstract class BootCompletedReceiver extends BroadcastReceiver { /** * Do not override */ @Override public void onReceive(Context context, Intent intent){ if(isBootCompleted(context, intent)){ onBootCompleted(); } } /** * This method is called when the BroadcastReceiver is receiving * {@link Intent#ACTION_BOOT_COMPLETED}. */ public abstract void onBootCompleted(); /** * @param context * @param intent * @return true if intent's action equals {@link Intent#ACTION_BOOT_COMPLETED}, otherwise false */ public static boolean isBootCompleted(Context context, Intent intent){ if(context == null){ throw new IllegalArgumentException("Context must not be null."); } if(intent == null){ throw new IllegalArgumentException("Intent must not be null."); } String action = intent.getAction(); return Intent.ACTION_BOOT_COMPLETED.equals(action); } }
おまけ: テスト用 adb shell コマンド
adb shell am broadcast -a android.intent.action.BOOT_COMPLETED